Top 50 JavaScript Interview Questions
JavaScript is one of the most popular programming languages used for web development. Therefore, if you’re preparing for a job interview, it’s essential to be familiar with the most commonly asked JavaScript interview questions.
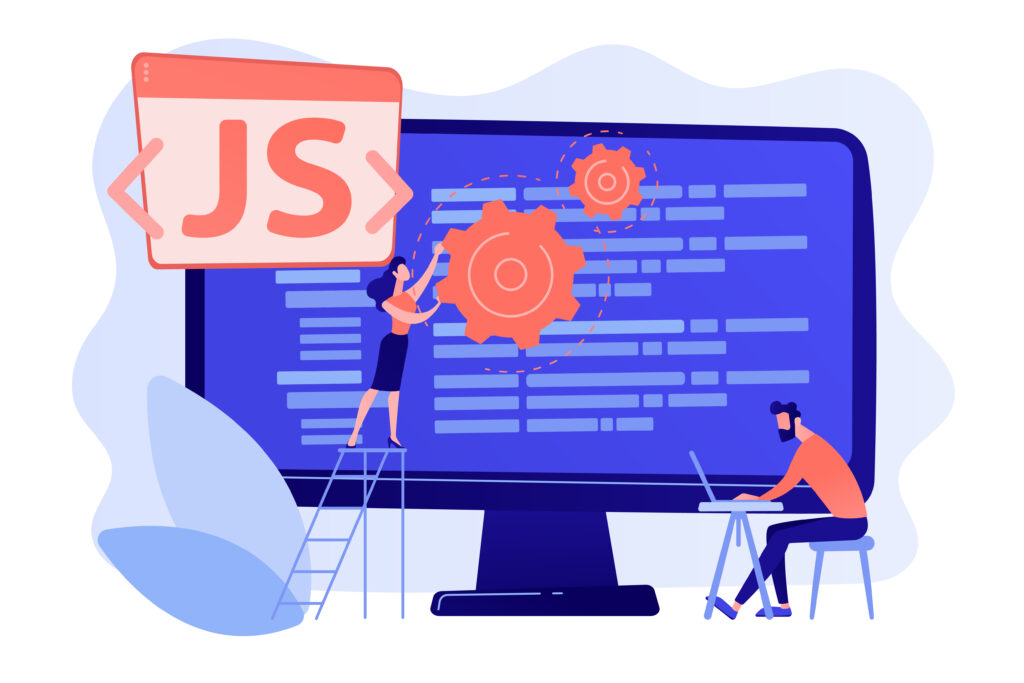
1. What is JavaScript?
Answer : JavaScript is a lightweight, interpreted programming language used to create dynamic and interactive effects within web browsers. It can be run both on the client-side (in the browser) and server-side (with Node.js).
2. What are the data types supported by JavaScript?
Answer : JavaScript supports seven primitive data types:
string
number
boolean
undefined
null
symbol
bigint
It also supports composite types such as objects and arrays.
3. What is a closure in JavaScript?
Answer : A closure is a function that retains access to variables from its outer scope, even after the outer function has returned. closures allow the inner function to continue accessing the outer function’s variables.
4. What is the difference between == and === in JavaScript?
- == compares values after performing type coercion, meaning it converts different data types to the same type before comparison.
- === compares both value and type without performing type coercion.
5. What are let, var, and const in JavaScript, and how do they differ?
- var: Declares a variable that has function scope or global scope if outside a function. Variables declared with var are hoisted.
- let: Declares a block-scoped variable. It doesn’t allow hoisting in the same way.
- const: Declares a block-scoped variable that cannot be reassigned, but its properties (for objects) can be mutated.
6. What is hoisting in JavaScript?
Answer : Hoisting is JavaScript’s default behavior of moving variable and function declarations to the top of their scope before execution. However, only the declarations are hoisted not the initializations.
7. Explain the concept of event delegation in JavaScript.
Answer : Event delegation allows a parent element to handle events from its child elements. this reduce memory usage by allowing one event handler to manage all child elements.
8. What is the this keyword in JavaScript?
Answer : This keyword refers to the object that is executing the current function. the value of this keyword changes depending on where and how the function is called.
9. What is the difference between call(), apply(), and bind() methods in JavaScript?
- call(): Invokes a function with a given this context and individual arguments.
- apply(): Invokes a function with a given this context, but accepts arguments as an array.
- bind(): Returns a new function with the this value set to the provided context and any arguments pre-set.
10. What are arrow functions, and how are they different from regular functions?
Answer : Arrow functions are a short syntax for writing functions. they do not bind their own this and arguments, which makes them suitable for non-method functions. they also do not have prototype.
11. What is the use of async and await in JavaScript?
Answer : Async and await allow asynchronous code to be written in a more synchronous-looking fashion. the async keyword makes a function return a promise and await pauses the execution until the promise resolves.
12. What is the difference between synchronous and asynchronous programming in JavaScript?
- Synchronous programming: Code is executed sequentially, with each step waiting for the previous one to complete.
- Asynchronous programming: Code runs independently, allowing other operations to continue while waiting for a response (e.g., fetching data from an API).
13. What is the event loop in JavaScript?
Answer : The event loop is the mechanism that handles asynchronous operations in JavaScript. It continuously checks the message queue and executes tasks in the queue when the call stack is empty.
14. What is NaN in JavaScript?
Answer : NaN stands for “Not-a-Number.” It is a special value returned when a mathematical operation results in undefined or unrepresentable value.
15. How does setTimeout() work in JavaScript?
Answer : setTimeout() sets a delay for the execution of a function after a specified time. It executes the code asynchronously after the delay.
16. What is the difference between null and undefined?
- null: It is an assignment value representing the intentional absence of any object value.
- undefined: It means a variable has been declared but not yet assigned a value.
17. What are promises in JavaScript?
Answer : Promises represent the eventual completion (or failure) of an asynchronous operation and its resulting value. they have three states: pending, fulfilled, or rejected.
18. What are callback functions in JavaScript?
Answer : A callback function is a function passed as an argument to another function, to be executed after the completion of the previous function.
19. What is the difference between deep copy and shallow copy in JavaScript?
- Shallow copy: Copies only the references of nested objects.
- Deep copy: Copies all objects and their references, creating a completely independent copy.
20. What is a prototype in JavaScript?
Answer : A prototype is an object from which other objects inherit properties and methods. every JavaScript object has a prototype and this prototype can be used to add new properties or methods.
21. What are higher-order functions in JavaScript?
Answer : A higher-order function is a function that either takes another function as an argument or returns a function as a result.
22. Explain the concept of rest and spread operators.
- rest operator (
...
): Collects all remaining elements into an array. - spread operator (
...
): Spreads the elements of an array into individual elements.
23. What is the difference between forEach() and map()?
- forEach(): Executes a function on each element of an array but does not return a new array.
- map(): Executes a function on each element and returns a new array with the results.
24. What are IIFEs (Immediately Invoked Function Expressions)?
Answer : IIFEs are functions that are executed immediately after they are defined. they are useful for creating a private scope to avoid variable collisions.
25. What is the purpose of object.freeze() in JavaScript?
Answer : object.freeze() prevents an object from being modified (adding, removing, or altering properties).
26. What is the use of JSON.stringify() and JSON.parse()?
- JSON.stringify : Converts a JavaScript object into a JSON string.
- JSON.parse() : Converts a JSON string back into a JavaScript object.
27. Explain the difference between Array.slice() and Array.splice().
- slice() : Returns a shallow copy of a portion of an array, without modifying the original array.
- splice() : Adds/removes elements to/from an array, modifying the original array.
28. What is the difference between null and undefined?
- null : Explicitly indicates the absence of a value.
- undefined : Means the variable has been declared but has not yet been assigned a value.
29. What is an arrow function in JavaScript?
Answer : Arrow functions provide a more concise syntax for writing functions. they do not have their own this binding, making them useful for maintaining the current context of this in callbacks and methods.
30. What is event bubbling in JavaScript?
Answer : Event bubbling is the process where an event triggered on a child element propagates up through its parent elements until it reaches the root element.
31. What is strict mode in JavaScript?
Answer : Strict mode is a feature that enforces stricter parsing and error handling in JavaScript code, making it easier to write more secure and optimized code.
32. What is a “pure function” in JavaScript?
Answer : A pure function is a function that, given the same inputs, will always return the same output and has no side effects.
33. How can you detect an object is an array in JavaScript?
Answer : Can use Array.isArray() to check if a value is an array.
34. What is the difference between localStorage and sessionStorage?
- localStorage: Stores data with no expiration time. data persists even after the browser is closed and reopened.
- sessionStorage: Stores data for the duration of the page session. data is cleared when the page or browser is closed.
35. What is use strict in JavaScript, and why is it used?
Answer : use strict is a directive introduced in ECMAScript 5 that allows to place a program or function in strict operating context. It helps catch common coding errors and prevents the use of unsafe features, making the code more secure.
36. What is memoization in JavaScript?
Answer : Memoization is a programming technique that optimizes performance by caching the results of expensive function calls and returning the cached result when the same inputs occur again.
37. What is the debounce function in JavaScript?
Answer : Debouncing ensures that a function is not called too frequently. It delays the execution of a function until a certain amount of time has passed since the last time it was invoked.
38. What is the throttle function in JavaScript?
Answer : Throttling limits the number of times a function is executed over time. Unlike debouncing, throttling ensures that the function executes at regular intervals, no matter how often the event occurs.
39. What is an async function in JavaScript?
Answer : An async function allows you to write asynchronous code that looks synchronous. It always returns a promise, and you can use the await keyword inside it to pause execution until a promise resolves.
40. What are JavaScript Promises?
Answer : A promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises have three states: pending, fulfilled, and rejected.
41. What is the event.preventDefault() method in JavaScript?
Answer : event.preventDefault() prevents the default action that belongs to the event. For example, it stops a form from submitting when the submit button is clicked.
42. What is event.stopPropagation() in JavaScript?
Answer : event.stopPropagation() prevents an event from bubbling up the DOM tree, stopping it from reaching parent elements.
43. How does JavaScript handle equality with objects?
Answer : JavaScript compares objects by reference, not by value. If two objects are not the same instance, even if they have the same properties and values, JavaScript considers them unequal.
44. What is Promise.all() in JavaScript?
Answer : Promise.all() takes an array of promises and returns a single promise. this promise resolves when all the promises in the array have resolved or rejects if any of the promises reject.
45. What is the difference between call() and apply() in JavaScript?
- call() : Calls a function with a given this value and arguments passed individually.
- apply() : Calls a function with a given this value and arguments passed as an array.
46. What is the purpose of the bind() method in JavaScript?
Answer : The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
47. What is the difference between synchronous and asynchronous JavaScript?
- Synchronous JavaScript: Code executes one statement at a time in sequence.
- Asynchronous JavaScript: Code allows other operations to continue running while waiting for an operation to complete, typically handled using callbacks, promises, or async/await.
48. How do you remove duplicates from an array in JavaScript?
Answer : remove duplicate by converting the array to a Set and then back to an array:
let array = [1, 2, 2, 3, 4, 4];
let uniqueArray = […new Set(array)];
49. What is destructuring in JavaScript?
Answer : Destructuring allows to unpack values from arrays or properties from objects into distinct variables. For example:
let {name, age} = {name: ‘John’, age: 30};
50. What is typeof in JavaScript?
Answer : The typeof operator returns a string indicating the type of the operand. It is used to check whether a variable is a string, number, object or another data type.
console.log(typeof 42); // “number”
console.log(typeof ‘hello’); // “string”
Top 50 Essentials JavaScript Interview Questions
Preparing for JavaScript interviews can be challenging but with the right knowledge of commonly asked JavaScript interview questions, you’ll be ready to tackle any technical challenge.